Creating scalable web applications is not so much a question of well-designed architecture, but also the appropriate tool set. There are thousands of modules in the Node.js ecosystem, but it is not all of them that are equally well-equipped to handle large loads and become an Ideal Node Module Set.
Why Module Selection Is of Crucial Importance?
Node.js is most famous for the asynchronous style of programming and ability to deal with thousands of simultaneous connections. Nevertheless, how well this task runs depends strongly upon the used libraries and utilities. The wrong modules’ selection could lead to:
- slower performance due to badly optimized queries,
- security holes because of unused code with vulnerabilities,
- cognitive difficulty with maintenance and new version installing after some time.
How Does Modules Affect Scalability?
As the load on a system increases, it is necessary that its components are able to handle the increased requests without losing performance and reliability. Some modules, such as Express.js, offer flexible control over HTTP requests, and PM2 makes scaling server processes possible. Using the right tools right from the beginning reduces technical debt and simplifies further development of the project.
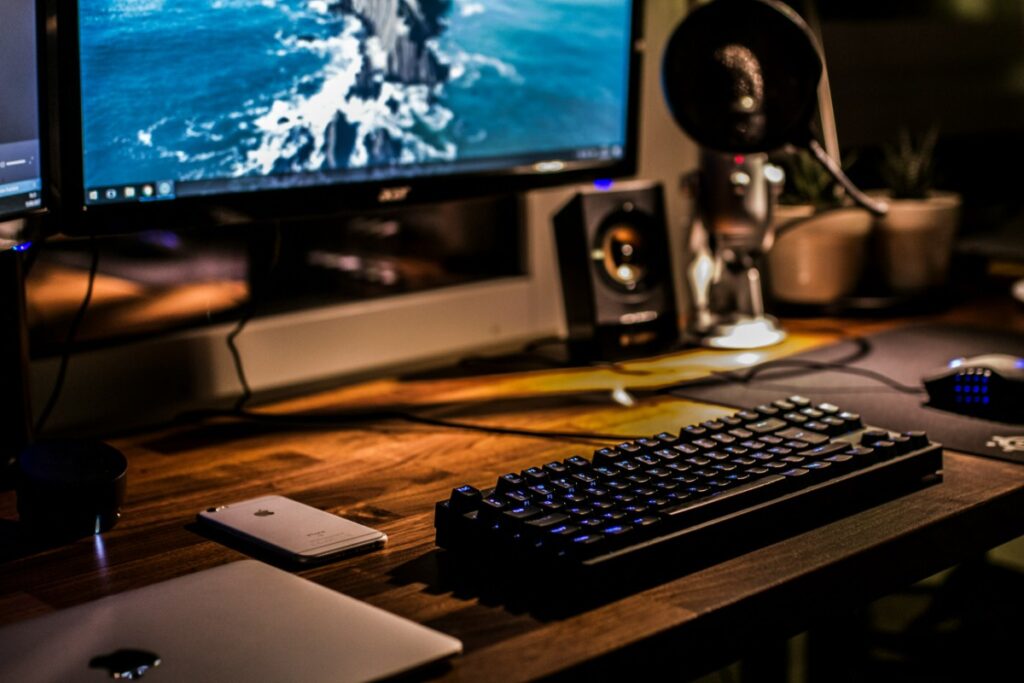
In this article from Celadonsoft, Node.js development company by link https://celadonsoft.com/node-js-development-company, we will break down the key criteria for selecting modules, review popular solutions for scalable applications and give recommendations on how to integrate them. Not only will this help you choose the most appropriate libraries, but also lay the basis for future development of your project.
Key Criteria for Selecting Modules for Scalable Applications
Deciding the right set of modules for Node.js is one of the most important aspects of building scalable web applications. Mistakes at this stage can lead to problems with performance, security, and system support in the long run. Let’s look at the key criteria that will help you select the best practices for your project.
1. Performance and Efficiency
Each Ideal Node Module Set you add to the stack has an impact on the speed of request processing, resource consumption, and scalability of the application. For example, using lightweight and optimized libraries (like Fastify instead of Express.js) can significantly improve server speed.
What is important to consider?
- Speed of operations execution. How fast does the module process request?
- Optimization of memory usage. Are there no memory leaks when running for long periods of time?
- Support for asynchrony and multithreading. For example, does the module support worker-threads to improve performance?
2. Community Support and Activity
Libraries that have a large and active community not only develop faster, but are also less likely to contain critical vulnerabilities. If a module is actively updated and discussed on GitHub, Stack Overflow, and other platforms, that’s a good sign.
How do I check for activity?
- The number of stars and forks on GitHub. Popular libraries tend to have scores in the thousands.
- Frequency of updates. If the last commit was several years ago – most likely the library is outdated.
- Responses to questions and bug fixes. The faster developers respond to issues, the higher the probability that the module will work stably in production.
3. Compatibility and Integration
Even if an Ideal Node Module Set looks powerful and promising, it’s important to consider how compatible it is with other tools in your project. For example, ORM Sequelize works great with PostgreSQL, but if you need MongoDB, Mongoose is worth considering.
What to look for?
- Compatibility with the version of Node.js used in the project.
- Availability of documentation and examples on integration with other libraries.
- TypeScript support (if you use it).
4. Security and Updates
Every external package is a potential source of vulnerabilities. Using unsupported or poorly secured modules can lead to serious security issues.
How to check security?
- Running dependencies’ analysis using npm audit.
- Using a proven security tool such as snyk or OWASP Dependency-Check.
- Read changelog before upgrading to understand what fixes and changes are being made to the module.
Overview of Approved Modules
Celadonsoft: “A module selection is the key to scalability and stability of Node.js applications. There are a multitude of tools for developers at their disposal, but not every tool can be applied to applications with a large load.” Let us examine the most critical modules that will help you create a strong, adaptive, and properly scalable architecture.
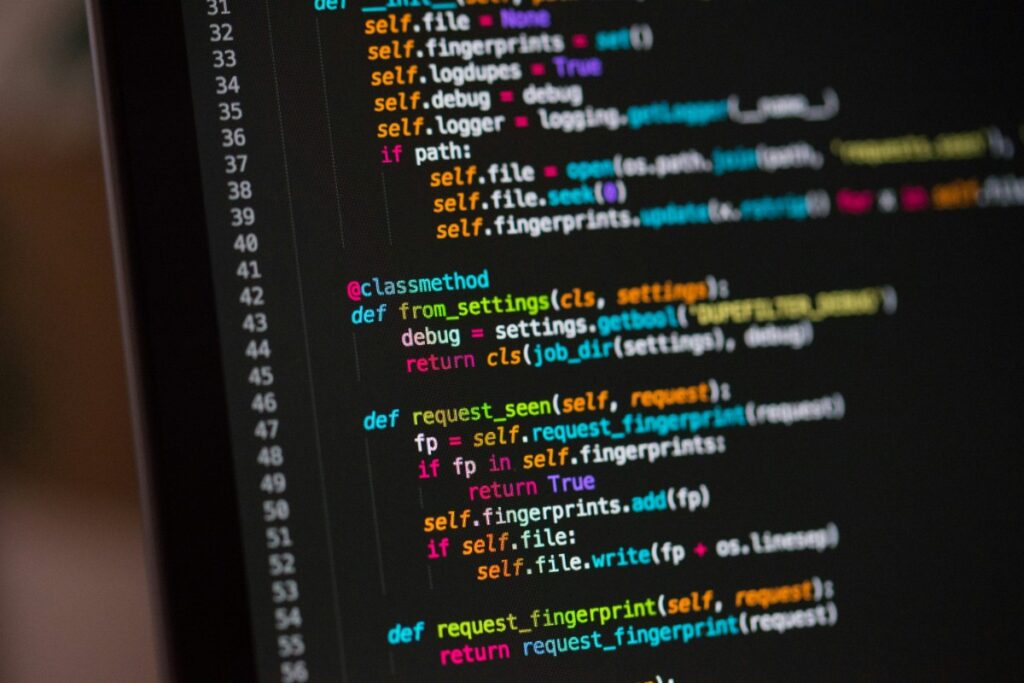
Express.js – Minimalism and Flexibility on the Server Side
Express.js is one of the most popular frameworks to create server-side applications on Node.js. Light, flexible and allows you to develop APIs and web applications very quickly.
Why Express.js?
- Extensible yet minimalistic – easy to shape to project needs.
- Rich middleware ecosystem that streamlines development.
- Performance-intensive when processing requests.
Express is ideal for building REST APIs and can also be used as a base layer for complex, scalable applications.
NestJS – Modularity and Strict Architecture
NestJS is a robust framework, TypeScript-based, inspired by the architectural standards of Angular. It’s ideal for building scalable server-side applications.
The key benefits:
- A clean modular structure which is convenient while working with huge projects.
- Native support for GraphQL, WebSockets and microservices.
- Compatibility with many ORMs like TypeORM and Sequelize.
NestJS simplifies working with large projects with robustly organized code and reproducible architecture.
Socket.io – Two-Way Real-Time Communication
Real-time data exchange (e.g. in chat, notification system or online game) is what your application requires? Socket.io is the solution.
What are the strengths of Socket.io?
- WebSockets and fallback feature for support of all browsers.
- Low latency data exchange.
- Easy event setup and seamless integration into Express/NestJS.
This Ideal Node Module Set is the de facto industry standard for maximum performance and reliability in real-time solutions.
Sequelize – ORM for Easy Database Interaction
You can even use Sequelize, a powerful Node.js ORM, for easy interaction with databases without tedious SQL queries.
Sequelize benefits:
- Multi-DBMS support (PostgreSQL, MySQL, MariaDB, SQLite, Microsoft SQL Server).
- Data model support through objects and associations.
- Migration and validation through built-in features.
ORM simplifies database work, especially in big projects where code readability and maintainability are important.
PM2 – Process Management and Auto-Restarting
PM2 is a process manager that simplifies the deployment and running of Node.js applications in production.
Why PM2?
- Auto-restarting applications upon crash.
- Distribution of loads between processes to make optimal use of server resources.
- Logging and monitoring tools.
PM2 is especially suited for applications running in high-availability configurations because it keeps downtime at a minimum and operation simple.
Practical Tips to Merge Modules
Celadonsoft: “Choosing the right modules is only half the battle. To get maximum scalability and stability of the web application, it is necessary to merge them correctly into the project.”
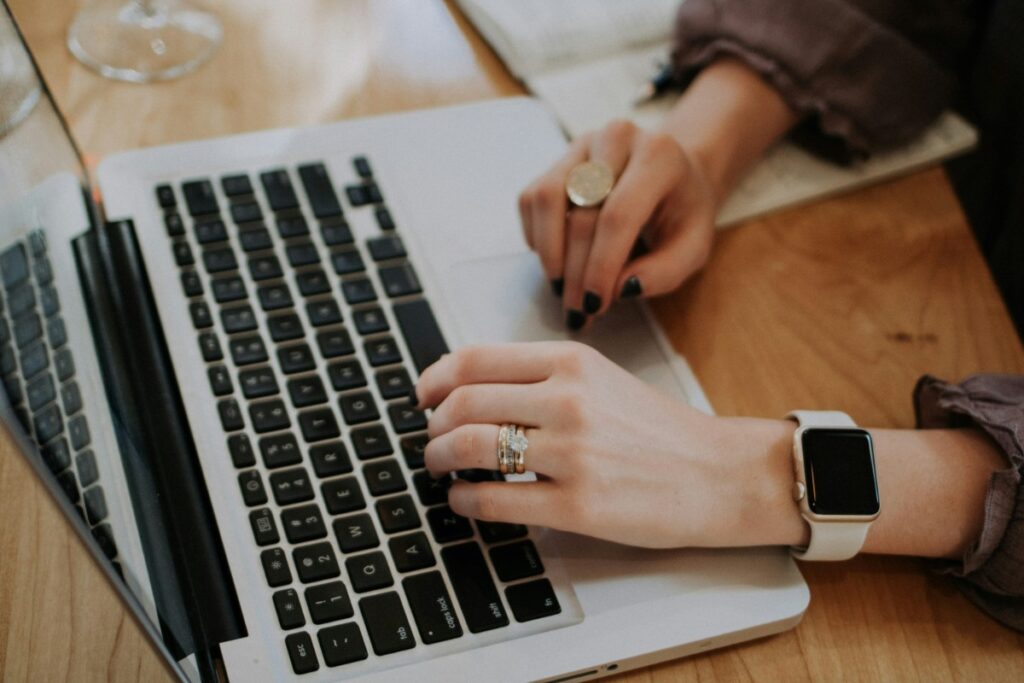
Project Structuring
Good architecture makes it easier to scale and keep code. With an example:
- Modular approach. Separate business logic, routes, and services into separate modules. This will ease refactoring and introducing new features.
- By using MVC or Hexagonal architecture. This will ensure that the business logic is independent of the interface and database.
Testing and Debugging
Code executed perfectly today can break when the load grows. Prevent this:
- Use Mocha or Jest for testing of API and business logic. This will catch bugs early.
- Use stress testing (k6, Artillery). This allows one to estimate what the system would do under massive loads.
Monitoring and Logging
The scalable application should be transparent. Consider the following:
- Logging. Use Winston or Pino for central log aggregation.
- Monitoring. Performance metrics can be monitored with tools like Prometheus and Grafana to watch for bottlenecks.
- Tracing. There can be analysis with OpenTelemetry with a microservice architecture.
Finalization
Choosing the ideal set of Node.js modules is a trade-off between performance, flexibility, and integration ease.
Best options:
- Define the project’s architecture. The more complex the app, the more important it is to have its infrastructure in place properly.
- Choose modules with an active community and regular updates. This will reduce security threats and ease support.
- Invest in testing and monitoring. Even top modules won’t keep you out of trouble if you can’t see what they’re doing under load.
- Use caching and load balancing. This will prevent servers from being swamped as more and more users hit the site.
Proper module selection is not merely about the speed of development, but also about long-term project viability. Organizations that make good choices about tools gain value through scalability and stability of web applications.